WordPress themes control how WordPress displays content. An incorrectly coded WordPress theme can cause a lot of problems ranging from display issues to incorrect template logic. Here are some common WordPress theme coding mistakes I’ve made myself or seen in other people’s code.
Ignoring the template hierarchy
One of the more common errors I’ve seen is the confusion between front-page.php
and home.php
. If you’re curious about the difference, check out my previous post about the topic. The TL;DR version is that you should provide a front-page.php
if you plan on supporting a static front page. This frees up home.php
or index.php
to be the “blog index”.
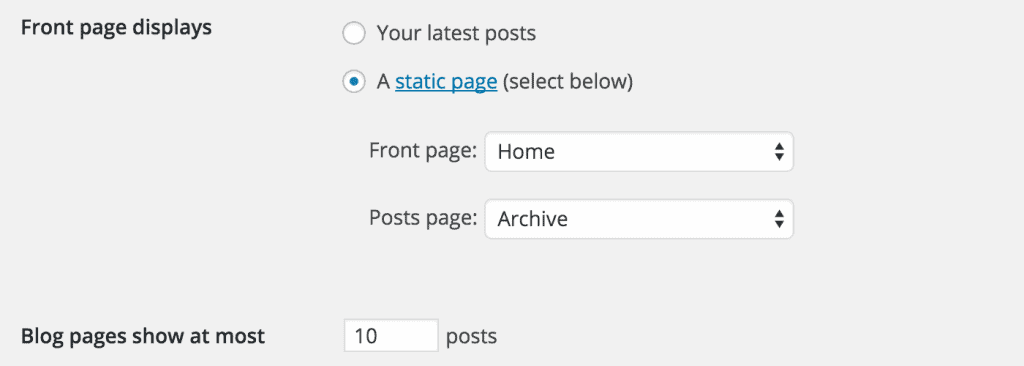
If you end up coding home.php
or index.php
to display a static front page, you’ve got a secondary problem. You might end up using a new template to actually display your blog index, so you turn to a custom template.
However, using a custom template means you are likely using a custom WP_Query
for your blog custom template, which in turn means that you will now need to code your own custom pagination, which can be a real pain.
Again, for more clarification, check out my earlier post about this.
Using reserved prefixes for custom templates
Custom page templates are a big reason why WordPress is so powerful. I’m not sure of the reason, but WordPress doesn’t provide a standard for page template filenames. However, it does provide a standard for regular page templates. Non-custom page templates use the prefix page-
.
If you’re coding up a custom page template, don’t name it page-my-custom-template.php
. WordPress will interpret it as a specialize page template in the form of page-{slug}.php
So what should you name the file? Use something that makes sense but isn’t a reserved keyword. I’ve tried a few different schemes but lately I like how The Theme Foundry names their templates: template-{name}.php
. WordPress.org also recommend putting them inside the page-templates
folder.
Not properly enqueuing your scripts and styles
Don’t hardcode links to your stylesheets and JavaScript files in your header.php
. In other words, you shouldn’t see something like this:
<head>
<link rel="stylesheet" href="css/normalize.css">
<link rel="stylesheet" href="css/main.css">
<script src="js/vendor/modernizr-2.8.3.min.js"></script>
</head>
There are a few reasons why you shouldn’t do this. Since WordPress is a platform, it involves themes and plugins that need to add their own CSS and JavaScript libraries, and they need to add it via the wp_head
function. If you hardcode your CSS and JavaScripts, there is no way to change the order in which they are loaded in case there are conflicts.
The proper way to add CSS and JavaScript files is to register and enqueue them. This gives theme and plugin developers a predictable way to call and inject CSS and JavaScript files. The WordPress Codex provides an example on how to enqueue your styles and scripts:
/**
* Proper way to enqueue scripts and styles
*/
function theme_name_scripts() {
wp_enqueue_style( 'style-name', get_stylesheet_uri() );
wp_enqueue_script( 'script-name', get_template_directory_uri() . '/js/example.js', array(), '1.0.0', true );
}
add_action( 'wp_enqueue_scripts', 'theme_name_scripts' );
Check out the tutorial on WPBeginner for an overview.
Trying to be a plugin
The WordPress Theme Review Guidelines state clearly:
Don’t do things in a theme considered plugin territory.
What is considered plugin territory? A good rule of thumb is if your site loses functionality when you switch themes, then that function belongs in a plugin.
A good example of plugin territory is custom post types. If you’re creating a church theme that supports the sermon post type, then that post type should persist even if the user switches away from your theme. Imagine the surprise of the user when all of their sermons disappear. (Yes, it’s still in the database, but most users don’t know that)
Not using version control
This isn’t exactly a theme-specific problem, but I have inherited themes that don’t use version control and the author ended up creating multiple copies of different files. I would see files like style-old.css or functions-old.php. This clutters the theme files and doesn’t give you much information on what was changed between the new and old versions of the files. With version control you have a history of changes and can revert to a certain point in that history.
Interesting stuff, David! One of my pet peeves is when I’m trying to look at a site and it doesn’t display properly on my phone or iPad and I have to enlarge it to be able to read it and keep moving back and forth because it won’t fit on screen. 🙁 You know it has to be something super important for me to do that, or forget it, I close the site as soon as I see there is s problem.
Poor code quality is a major contributing factor to poor WP theme quality. Lack of spacing and inconsistent styles makes your website’s code harder to load and run. Besides, it’s just messy. By looking at the javascript files included in some of the best-selling themes at http://www.templatemonster.com/wordpress-themes.php or http://themeforest.net/category/wordpress, you might notice how well written/organized they are. Some WP theme developers should definitely work on getting their coding standards improved.
Hey David,
Great article, and while I knew most of these tips there are a still a few I didn’t know about. One thing I see on some new (and maybe even older) WordPress site is people don’t disable/remove the meta admin widget from their sidebar. No reader/viewer/client/customer, etc needs to see a link for you to log into your WordPress dashboard when they got to your site. That tab is completely useless (just go to yoursite.com/wp-admin) and should be removed as soon as your site is active.
Hi Shivam,
Thanks! Yes, that’s a good tip. I’m not a fan of the meta admin widget!
I may suggest to visit http://howtotech.org/how-to-avoid-wordpress-design-mistakes-for-beginners